BOOK TRAILERS
You May Be Born In One Place...
Making An Earthly Impact
Not Let What You See Determine...
Finished Accomplished
Now You Can Succeed
Let God Transform YOU
Let Not Your Beginning Determine Your End
According To The Abilities He Created In You
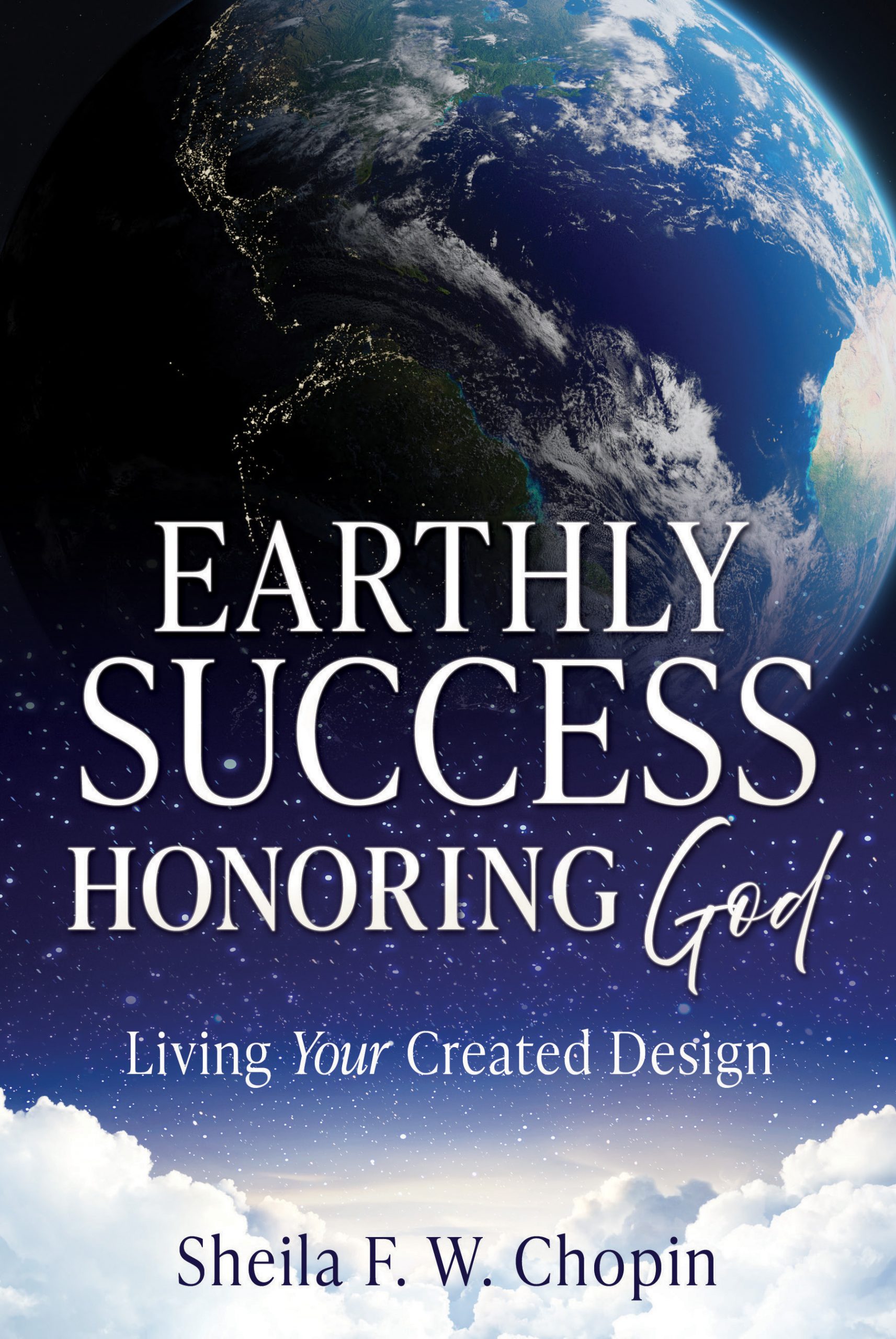
Sheila F.W. Chopin
Sheila Chopin is a wife and mother of two incredible sons. Her life has been dedicated to God, family, Christian ministry, mentoring, and engineering. Her start was in a small country town, working on the farm. She learned people were valuable despite their beginnings and location. Sheila worked as an engineer and manager in aerospace and semiconductors for over thirty-seven years. Her business travels involved North America and beyond to Asian and European countries, collaborating with persons worldwide.
Sheila has co-authored thirty technical publications and released sixteen US Patents. Her public speaking includes Christian, Technical, and University. Her Christian writings aim to encourage people everywhere to truly experience God's amazing love, living their created design.
TESTIMONIALS
"This book is so inspiring and well written. It feels like God hugging us through the author's writing. Loving it! "
- Cassandra Thomas (Amazon.com)
"A HOPEFUL book full of practical and encouraging WISDOM about how to live an abundant life -- to have VICTORY in life. "Earthly Success Honoring God" uses God's word and scripture as the foundation for all of its advice.
This book simply, but profoundly, explains the nature of who God is and shows readers how TRUSTING GOD and having faith in HIM can lead us to living out our created design.
This is the best kind of "self-help" book out there because it curates the TREASURE that is the WORD OF GOD. For people who haven't read the entire Bible or often get lost reading its text, they will get a focused and generous portion of God's promises to mankind. How RICH and POWERFUL God's own words are.
"...the word was GOD..." (John 1:1).
The author demonstrates what has been helpful in her personal experience as a witness to God's grace in her life and the lives of those around her. She insightfully presents passages from the Word to point readers to a transcendent thesis -- receiving success in this life by HONORING God, SEEKING HIM FIRST.
It is abundantly clear that the author has a close, intimate relationship with the Lord and has been tasked with writing a book to help guide people and followers of Christ especially. The posture of the author's heart is one of humble love with a desire to instruct, encourage, and BENEFIT those who read.
In a world full of noise and thoughtless information, this BOOK is of REAL VALUE and SUBSTANCE. One worthy of TIME and multiple readings. Timeless in every generation and for people of all nations.
Please do yourself a favor and buy this book for everyone you know!!! Read it for your own benefit and help others by gifting it.
Hebrews 4:12 - " For the word of God is living and powerful, and sharper than any two-edged sword, piercing even to the division of soul and spirit, and of joints and marrow, and is a discerner of the thoughts and intents of the heart.""
- Sunshine_Sparrow (Amazon.com)
FOREWORDS
Earthly Success Honoring God is an intimate exploration into the heart of God and His desire for His children to succeed in their lives. This book provides readers with profound insights and principles that can cultivate a mindset of determination, enabling them to grow in faith and finish well. Reading this book has profoundly impacted me. It has inspired me to seek God more fervently than ever before and move forward confidently. I am now committed to writing my vision and trusting that God's will be done in my life and the lives of my loved ones. While we all aspire to achieve "success," the success that comes with God, as the author beautifully illustrates, is far greater than anything we could achieve on our own. The author thoughtfully includes scriptures for successful living, offering readers valuable passages to review and meditate on. After reading this book, I am truly excited about the new journey I am about to embark on. I have faith that God's perfect will shall prevail, leading to a successful and fulfilling outcome in the end.
What a powerful book! Just two pages in, and tears welled up in my eyes. It is a remarkably accurate reflection of a life testament of living victoriously as God intended. As both a highly respected leader at work and a dear sister in Christ, Sheila has always inspired me. Her discipline in spending quiet time with God every morning is remarkable. This book will help translate your faith into excellence in your work. Earthly Success Honoring God shares the secrets of success, which will undoubtedly be a great blessing to everyone in the world and help make this world a better place.
Throughout the 30 years of knowing the author, Sheila has encouraged me to focus on understanding God’s plans for my life, deepen my relationship with Him, and learn how to pray listening for His guidance. She has encouraged me by word and deed of the importance of being fruitful, productive, and the best I can be. Her influence has been an extraordinary blessing in countless ways, leaving an indelible mark on my life.
As you delve into "Earthly Success Honoring God: Living Your Created Design," prepare to embark on a transformative journey. This book is a guide and a beacon of inspiration that will ignite your passion for living a life of purpose, honor, and excellence. Sheila’s wisdom and experiences will challenge you to embrace your divine design and live remarkably, accomplishing the extraordinary works that God has preplanned for you. But you must tap into Him and the things of God, which includes His plans and purposes for your life. This book will help you do that, indeed! May this book richly bless your life and empower you to shine brightly in all you do.
Join Sheila Faye Chopin on this journey that explains how hard work while walking with God yields success! I have observed her as a mentor, author, public speaker, family member, friend, and professional patented engineer for over 35 years. This godly woman added value to others, including the corporate world, as she carried her Christian values to her international workplace.
Her transformational leadership and Christian teachings have touched lives worldwide while she aligned her faith with her profession. Your success is waiting for you. Various examples to achieving that success is conveyed in this gem of a book.