Create Static Members
Until now, we have created instance members of classes where every object that is instantiated will have its OWN UNIQUE COPY of all of the properties and methods of that class. However, you can create static (or shared) members where a property or method is SHARED ACROSS all objects of that class.
EXAMPLE:
Before:
REPLACE LATER WITH OWN SCREENSHOT
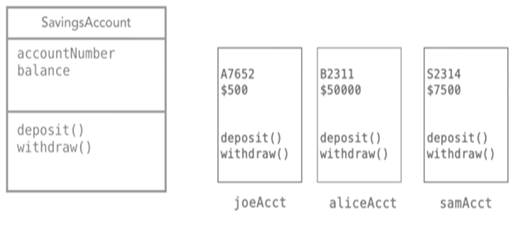
NOTE: Multiple objects are instantiated that all have their OWN COPIES of the properties and methods of that class.
After:
However, if you realized that all of the objects need an interest rate, you could define it as a regular property (an instance variable) where every object has its own copy of interest rate.
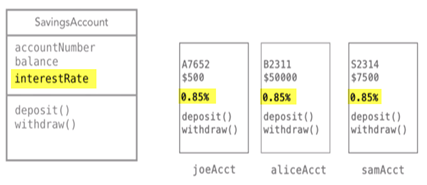
However, if you want the interest rate to be shared across all saving account even though it might change but not vary from account to account, there is no need to have multiple copies of the property. We can define the interestRate as a static variable (property) which is sometimes called a class-level variable or shared variable as oppose to an instance-level variable where only ONE COPY is shared across all objects.
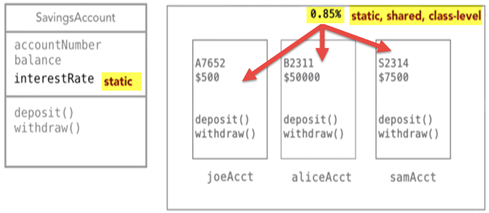
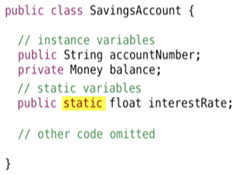
NOTES:
- Notice the key word static was added to the interestRate where it belongs.
- The static key word means that there is ONLY ONE COPY of that property or method.
- The term static does NOT mean constant. As mentioned earlier, it can still change but there is only ONE COPY across all objects.
- It is important to remember that when you access an instance-level variable, you access it by using the object name.
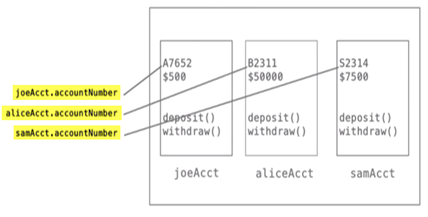
- But, if you want to access a static (or shared) variable, you access it by using the class name and not the name of any instance of this class regardless of the number of objects or even if there is no object created.
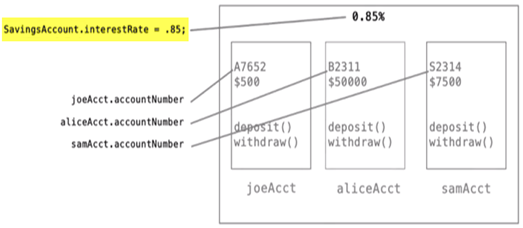
- Even if you don’t instantiate any objects from this class, you can still use any variables defined as static. So, you could set the interest rate for this class BEFORE creating any object of this class.
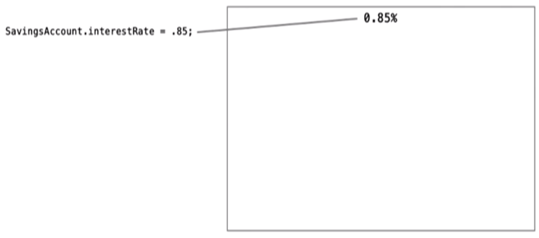
- You can also create static methods.
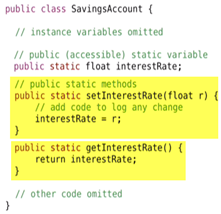
- Static methods can ONLY access static variables.
- You cannot write code in a static method to access instance-level variables.
- Notice that static methods (see highlights above) are used to get and set the interest rate. However, the interestRate static variable should be changed to private—you would not want another object to change the interest rate.
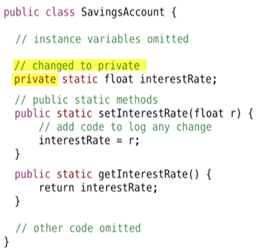
- Static does not affect visibility. So, the class name along with the static class-level method can be used to SET the interest rate.
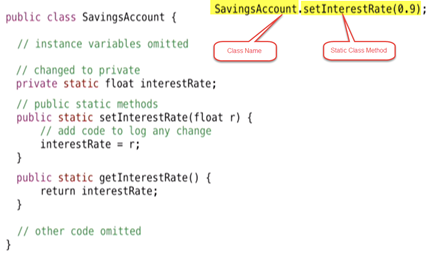
- In a UML class diagram, static members (property or methods) are typically shown with an underline so that you can recognize that they exist at the class-level and not the instance-level.
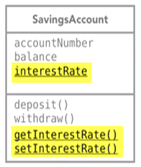
- Most classes are defined as the instance-level variables, but class-level variables are useful when needed.