Create An Overload Constructor
You can have more than one constructor in a single class which is called OVERLOADING the class. If you want to “construct” a CUSTOMIZED object instead of a PRE-DEFINED object each time you create an object, you can create one or more constructor that you can pass arguments into it to customize the object.
REPLACE LATER WITH OWN SCREENSHOT
EXAMPLE:
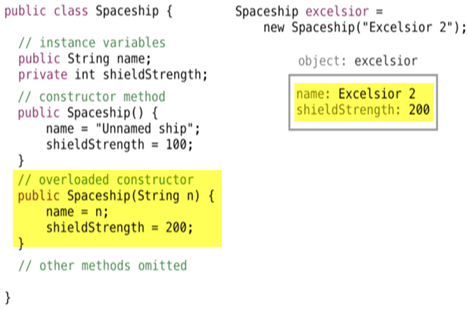
REPLACE ABOVE WITH MY CODE:
public class MyCustomBox {
// instance variables
public String height;
public String width;
public String depth;
// regular constructor method for 2D object
public MyCustomBox(String height, String width)
{
height = 100;
width = 100;
}
// overload constructor method for 3D object
public MyCustomBox(String height, String width, String depth)
{
height = 100;
width = 100;
depth = 100;
}
// Other methods here...
}
Now, create two pre-defined objects (a 2D and a 3D object) from the SAME class:
MyCustomBox my2DBox = new MyCustomBox();
MyCustomBox my3DBox = new MyCustomBox();
NOTES:
- If a class has more than one constructor, you can use any of the constructor method depending how you type the instantiation code.
- When a class is overloaded with constructors, it offers more flexible in passing data into the constructor before it is created.
- The UML class diagram would have multiple methods representing each constructor.
- When using a class with multiple constructors, it is important to select the CORRECT constructor method when creating an object that needs to be used by other objects with that constructor type.
- Typically, a method has a unique name within its class. However, it may have the same name if the method is overloaded. The compiler can distinguish between the method signatures to select the compile the correct method.
- All constructors in a class can be declared because they all have different number of arguments. The compiler can differentiates the number of arguments and serve up the correct constructor. You cannot write two constructors with the same number or type of argument for the same class. If you do, the compiler will throw a compile error.
- All classes will have at least one constructor. If you don’t EXPLITCILY create one, the compiler will AUTOMATICALLY create a default constructor that is called a no-argument constructor. It calls the class parent’s no-argument constructor or the Object constructor which does have a no-argument constructor if the class has no parent.
- You can use access modifiers in a constructor's declaration to control which other classes can call the constructor.
- Overloading a class with methods should be used only when needed because it makes the code harder to read.
REPLACE LATER WITH OWN SCREENSHOT
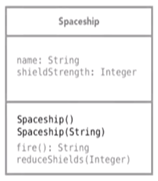